Auth in Flutter
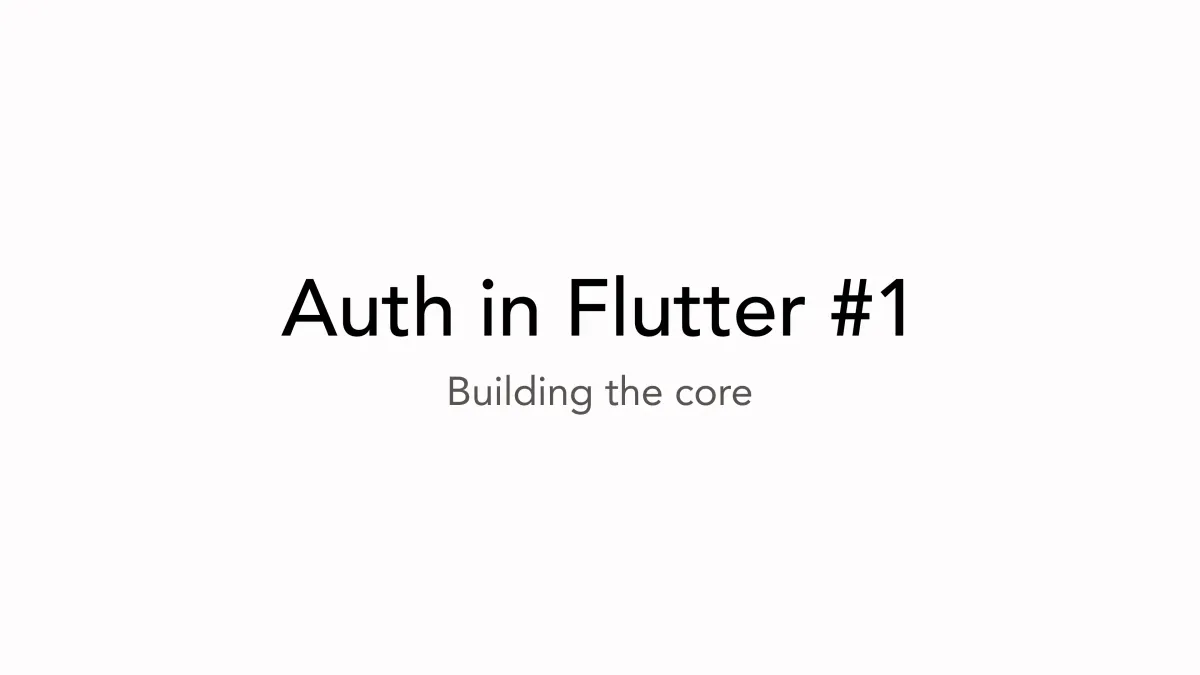
Authentication and authorization are crucial components of modern applications, ensuring that users can securely access and interact with the features and data they're entitled to. I'm starting a series of articles that dive deep into doing it right, with a focus on practical insights and essential theory. Let's tackle some key questions:
- Responding to Auth State Changes: How do we effectively monitor and react to changes in a user's authentication status?
- Creating an Auth Interceptor: How can we implement an authentication interceptor that incorporates token refresh and retry logic to handle failed requests seamlessly?
- Integrating Auth with Navigation: How do we tie authentication states to our navigation system, ensuring users are directed appropriately, and how do we leverage guards and deep links for a smoother user experience?
- App Initialization: What are the best practices for initializing an application with authentication in mind, ensuring a secure and user-friendly launch?
Final Result
Outline
Fundamentals
Authentication and authorization are fundamental security concepts that work hand-in-hand to ensure secure access to systems and resources. Here's an explanation of these concepts:
Authentication is the process of verifying the identity of a user, system, or entity before granting access to a system or resource. This can be accomplished by providing credentials, such as a username and password, biometric data (e.g., fingerprint, facial recognition), or other forms of identification. Authentication mechanisms can also involve multi-factor authentication (MFA), which adds an extra layer of security by requiring additional forms of verification, such as a one-time password or a physical token.
Authorization, on the other hand, is the process of determining the specific permissions, privileges, or access levels that an authenticated entity has within a system or resource. Authorization is based on predefined rules, policies, or roles that define what actions an authenticated entity is allowed to perform, such as reading, writing, modifying, or deleting data, executing specific functions, or accessing certain areas of a system.
The relationship between authentication and authorization can be summarized as follows:
- Authentication comes first: Before an entity can access a system or resource, its identity must be verified through the authentication process. This establishes trust and ensures that only legitimate users or systems can gain entry.
- Authorization follows: Once an entity's identity is authenticated, the authorization process kicks in to determine the specific actions or resources that the entity is permitted to access or perform within the system. This ensures that even authenticated entities can only access and perform operations based on their assigned roles, permissions, or privileges.
OAuth
OAuth (Open Authorization) is an authorization framework that enables secure, delegated access. It allows users to grant third-party applications limited access to their resources on a service without sharing their passwords, providing a standardized and secure approach to authorization across numerous systems.
OAuth defines the following key entities:
- Authorization Server: The server responsible for issuing access tokens to clients after successful authentication and authorization. It acts as a trusted intermediary between the resource owner, client, and resource server.
- Resource Server: The server that hosts the protected resources (data, APIs, etc.) and requires authorized access via access tokens.
- Resource Owner: The entity (typically a user) capable of granting access to their protected resources hosted on the resource server.
- Client: The application that requests access to protected resources on behalf of the resource owner and with their authorization.
The OAuth flow involves the client obtaining an access token from the authorization server after the resource owner grants permission. The client then uses this access token to make authorized requests to the resource server on the resource owner's behalf. Additionally, OAuth introduces the concept of refresh tokens, which are special tokens used solely to obtain new access tokens without requiring the resource owner's involvement.
By separating the roles of the authorization server and resource server, OAuth ensures that sensitive credentials, such as usernames and passwords, are never shared with third-party applications. This enhances security and provides a standardized and scalable approach to authorization across diverse systems and applications.
+--------+ +---------------+
| |--(A)------- Authorization Grant --------->| |
| | | |
| |<-(B)----------- Access Token -------------| |
| | & Refresh Token | |
| | | |
| | +----------+ | |
| |--(C)---- Access Token ---->| | | |
| | | | | |
| |<-(D)- Protected Resource --| Resource | | Authorization |
| Client | | Server | | Server |
| |--(E)---- Access Token ---->| | | |
| | | | | |
| |<-(F)- Invalid Token Error -| | | |
| | +----------+ | |
| | | |
| |--(G)----------- Refresh Token ----------->| |
| | | |
| |<-(H)----------- Access Token -------------| |
+--------+ & Optional Refresh Token +---------------+
To gain a deeper understanding of OAuth, I highly recommend reading the RFC 6749 specification.