Recommended Libraries for Dart & Flutter
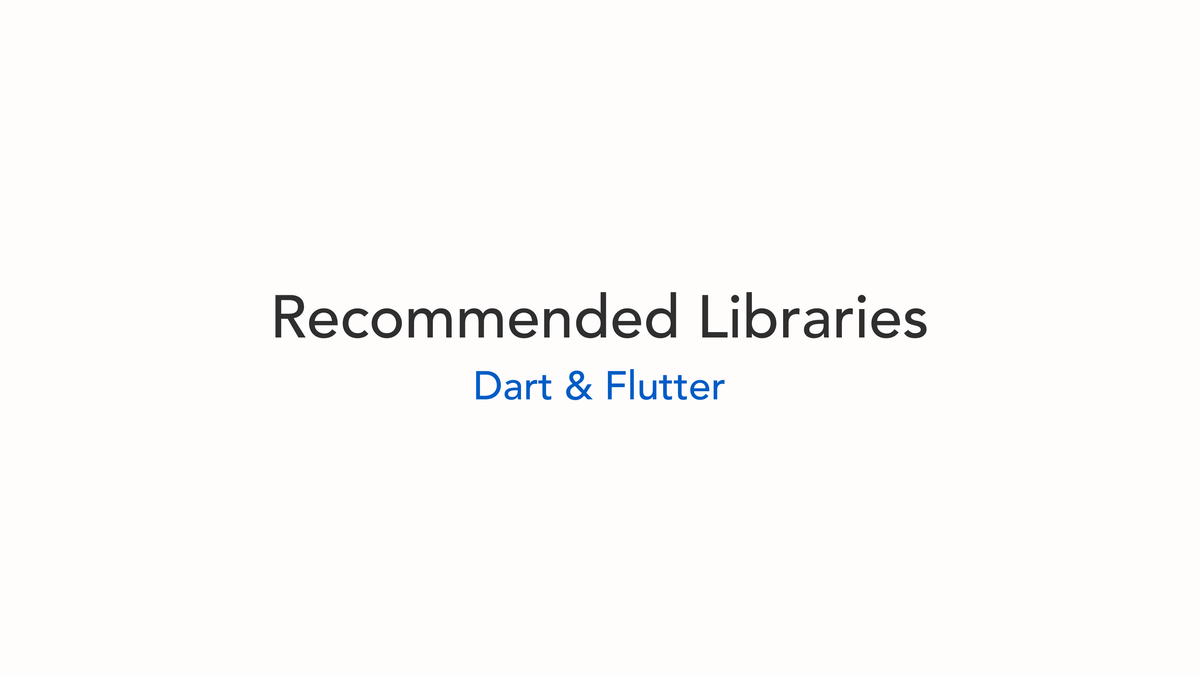
This page lists recommended packages and plugins that have been tested and approved for production use. In case you're interested in libraries that are not recommended go to Avoid These Dart Libraries.
Built-in
Essential Dart libraries providing foundational functionality across all Flutter projects.
- collection: Contains utility functions and classes in the style of
dart:collection
to make working with collections easier. It contains ways to shuffle aList
, do binary search on a sortedList
, and various sorting algorithms. The package also provides a way to specify the equality of elements and collections. - math: Offers mathematical constants and functions, including trigonometry, exponential, and logarithmic operations.
- async: Provides utilities for handling asynchronous programming, including
Future
,Stream
, andCompleter
. - intl: Internationalization and localization support, including formatting dates, numbers, and messages.
- meta: Supplies annotations like
@required
,@immutable
, and@protected
, aiding in code readability and analysis. - path: Cross-platform utilities for manipulating and constructing file paths, like joining, splitting, normalizing, etc
- characters: Handles complex string manipulation, particularly for strings with special Unicode characters.
Utilities
Enhance and streamline your Flutter development with these utility libraries.
- flutter_native_splash: Generates native splash screens for iOS, Android and Web.
- path_provider: A Flutter plugin for finding commonly used locations on the filesystem. Supports Android, iOS, Linux, macOS and Windows.
- json_serializable: Automates the generation of code for serializing and deserializing JSON.
- url_launcher: Easily open URLs, email clients, or SMS apps from within your Flutter app.
- flutter_gen_runner: Generates code for assets, fonts, and more to improve type safety and reduce runtime errors.
- pigeon: Facilitates communication between Flutter and native code by generating type-safe platform channels.
- google_fonts: Enables the use of Google Fonts in your app, ensuring consistent typography across platforms. Supports HTTP fetching at runtime, ideal for development. Can also be used in production to reduce app size.
- permission_handler: Manages app permissions across platforms, simplifying permission checks and requests.
- share_plus: Allows users to share text, URLs, files, and more with other apps.
- flutter_local_notifications: Provides a cross-platform API for displaying local notifications in your app.
- flutter_blurhash: Displays blurred placeholder images while the full image is loading.
- sensors_plus: Interface with device sensors like the accelerometer, gyroscope, and magnetometer to gather real-time environmental data.
- image_picker: A Flutter plugin that enables users to pick images and videos from the device's gallery or camera. It also provides options for image cropping and video compression.
- file_picker: A versatile plugin for picking files of any type from the user's device, including images, documents, audio, and video files. It supports multiple file selection and provides detailed file metadata.
- web: Lightweight browser API bindings built around JS interop.
Network
Handle networking tasks and backend integration with these essential libraries.
- http: A simple yet powerful library for making HTTP requests and processing responses.
- dio: A powerful HTTP client for Dart, offering advanced features such as request/response interceptors, global configuration, file uploading/downloading, and support for handling complex networking tasks like retries, timeouts, and authentication.
- firebase: A suite of backend services from Google, offering real-time databases, authentication, analytics, and more.
Storage
Manage persistent local data effectively with these libraries.
- shared_preferences: Store simple data (key-value pairs) persistently across app launches.
- drift: A reactive persistence library that simplifies working with SQLite databases. Supports advanced features - transactions, schema migrations, complex filters and expressions, batch updates and joins. Also provides the ability to generate type-safe queries directly from SQL.
- sqflite: SQLite plugin providing robust local database management for storing structured data.
Streams
Harness the power of asynchronous data streams with these advanced libraries.
- rxdart: Adds reactive programming capabilities to Dart streams, offering a wide range of operators for stream manipulation.
- stream_transform: Utilities for transforming streams, enabling more complex and flexible stream-based workflows.
Animations
Create engaging and dynamic user experiences with these animation libraries.
- Lottie: Render Adobe After Effects animations natively in Flutter apps for visually appealing motion graphics.
- Rive: Design and integrate interactive and performant vector animations directly into your Flutter app.
- animations: A collection of pre-built animations, including transitions and other motion effects, to enhance your app's UI.
- flutter_animate: Provides a rich set of animations, easing functions, and custom transitions to enhance your app's UI.
Location
Essential libraries for building apps with location-based services.
- Geolocator: Access and manage the device's GPS location services, including geocoding and geofencing.
Backend
Build and manage server-side logic and web services efficiently.
- Shelf: A flexible web server middleware for Dart that allows the creation of modular web applications and APIs. This is preferred over serverpod, dart_frog, and others.
Application Performance
Monitor and improve your app's performance to ensure a smooth user experience.
- Sentry: Tracks errors and performance issues, helping you diagnose and fix problems in your Flutter app.
Media
Integrate audio and video features seamlessly with these media libraries.
- just_audio: A powerful audio player that supports playing from various sources, including local files and network streams.
- video_player: A plugin for playing video content from files or streams, supporting a wide range of formats.
Testing
Ensure the reliability of your app through comprehensive testing.
- mockito: A popular mocking framework that simplifies the creation of mock objects in your tests. Preferred over Mocktail as it provides a much more convenient and bug-proof API.
- clock: Provides a testable alternative to
DateTime.now()
andTimer
, making time-dependent code easier to test. - fake_async: Allows you to control time within your tests, facilitating the testing of time-dependent logic.
Feedback
Gather valuable user feedback directly within your app.
- feedback: A plugin to collect in-app user feedback, including screenshots and annotations, helping you understand user issues.
System
Gain deeper access to system-level features with these libraries.
- win32: Provides direct access to Windows API, enabling advanced native functionalities for Windows-based Flutter apps.
Game Development
Build 2D games and interactive applications with specialized game development libraries.
- flame: A lightweight game engine for creating 2D games in Flutter, offering components like physics, collision detection, and sprite rendering.