Authentication in Flutter with Supabase (OAuth included)
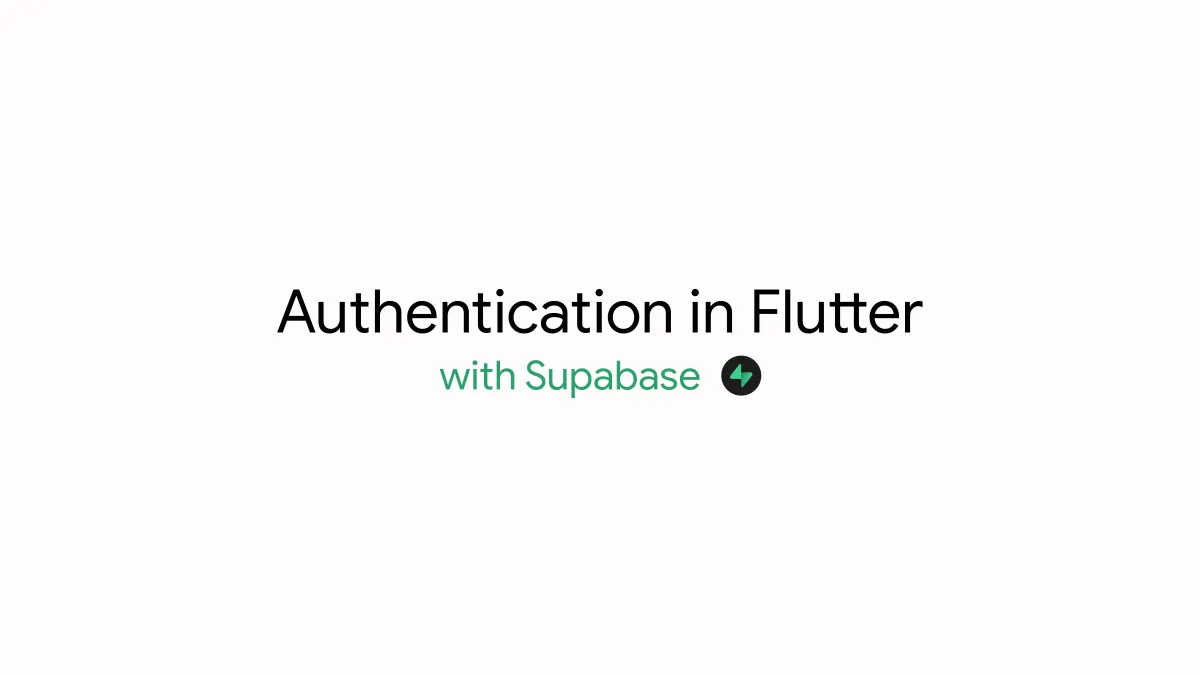
I’ve been working on a Flutter app for a while and recently decided to add authentication. Setting it up correctly is challenging, especially when it comes to managing backend infrastructure and administration. So, there are several common approaches to implementing authentication, including:
- Building a custom authentication service
- Using a cloud-based service like Auth0, Firebase, or Okta
- Deploying a self-hosted solution like Keycloak or Supabase
After carefully evaluating these options, I realized that creating a custom authentication service and managing the infrastructure would consume a lot of time — something I wanted to avoid, especially for an MVP.
This led me to look at pre-built solutions. I initially considered Firebase, Auth0, and Clerk, but found their pricing prohibitive and noticed that some aren’t fully supported in Flutter (for example, Firebase isn’t available on Desktop).
Then, I discovered Supabase, an open-source Firebase alternative that is written in pure Dart. In this article, I’ll cover how to implement Authentication (with OAuth via Google) using Supabase, along with setting up a robust architecture that avoids tight coupling. This is going to be useful even if you do not use Supabase as I’ll walk through creating guards, an authentication data provider, and a BLoC to manage the flow.
Outline
Auth Provider
Our journey starts with the data provider, the foundation of the app’s architecture. The data layer handles data exchange, specifically in this case, by communicating with Supabase. If you're not familiar with layered architecture, check out my Flutter App Architecture series.
Here’s the AuthProvider
interface: